Algorithm Assignment Help
1. What is an Algorithm?
An algorithm can be defined as a set of steps to accomplish a task. You might have an algorithm from getting from home to school, for making a grilled cheese sandwich or for finding what you’re looking for in a grocery store.
In computer science, an algorithm is a set of steps for a computer program to accomplish a task. Algorithm put a science in computer science. Finding good algorithm and knowing when to apply them will allow you to write interesting and important programs. Let us go through some examples now.
Consider how do you use a computer in a typical day, for example, you start working on a report and once you have completed a paragraph you perform a spell check. You open a spreadsheet application to do a financial projection. You want to buy a new car and open a web browser to perform the search operation. All of the above operations done by your computer consists of computer algorithms.
Algorithms are generally created independent of the languages, i.e. an algorithm can be implemented in more than one programming language.
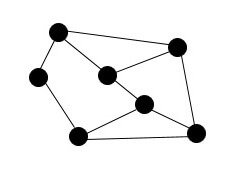
Figure 1: Demonstrating layout of interconnected steps in an algorithm.
2. Algorithms in Programming
We can think of a programming algorithm as a recipe that describes the exact steps needed by the computer to solve a problem or to reach a goal.
Algorithm Assignment Help By Online Tutoring and Guide Sessions at AssignmentHelp.Net
In computer language, the word recipe can be replaced by procedure and the word ingredient by inputs and you get to see the results which are called output. Once you convert your algorithm to the language computer understands, the computer would do it in the same way every time. However, it is important to note that a programming algorithm is not a computer code, it is written in simple English or the language the programmer understands.

Figure 2: Demonstrating layout of computer programming algorithm involving series of steps, inputs and output.
3. Methods of specifying algorithms
3.1 Pseudocode
In Pseudocode steps of algorithms are specified using essentially natural language of superimposed control structure. Let us go through the following example to calculate pay of an employee depending on the number of hours invested and rate of per hour.
Pseudocode example
{` BEGIN input hours input rate pay = hours * rate print pay END `}
3.2. Flowchart:
A Flowchart is a traditional graphical tool with standardized symbols which shows the sequence of steps in an algorithm. Let us go through the following flowchart to exhibit the previous example.
Flowchart example
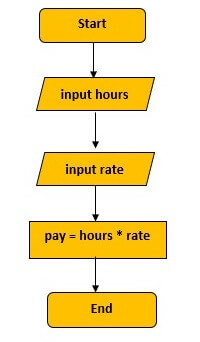
4. Properties of Algorithm
4.1 Finiteness
An algorithm should have an exact number of steps to be taken and should have an end as well.
4.2 Absence of Ambiguity
In an algorithm, every instruction should be precisely described and specified clearly.
4.3 Sequence of Execution
Instructions should be performed from top to bottom in an algorithm.
4.4 Input and Output
The unknowns defined in the problem should be specified clearly with the expected outcomes.
4.5 Effectiveness
The solution prescribed should be guaranteed to give a correct answer or expected results and the specified procedure should be faithfully carried out.
4.6 Scope Definition
The algorithm should be applicable to a specific problem or class of problem.
5. Steps to Design an Algorithm
5.1 State the problem/goal clearly
To solve a problem correctly it should be explained in detail and in clear terms.
5.2 Plan and write the logical order of instructions
The computer should follow the instructions in the same order as given by the programmer to perform a specific algorithm.
5.3 Write code for the program
Writing the programming statements in the desired languages.
5.4 Giving the program to the compiler
Typing the program or statements into the computer to make the code compile.
5.5 Running and debugging the program
After compiling the program, the phase to run the program comes. Check if you are getting a desired output. In case of an incorrect or ambiguous output trace back the possible error.
6. Symbols in a Flow chart
Usually the Flowchart of an algorithm is designed and identified using different symbols shown as above.
SYMBOL | NAME | DESCRIPTION |
---|---|---|
![]() | TERMINAL | Defines the start and end point of a flowchart. |
![]() | INITIALIZATION | The preparation or initialization of memory space for data processing. |
![]() | INPUT/OUTPUT | The inputting of data for processing, and printing out of processed data. |
![]() | PROCESS | Manipulation of data (assignments and mathematical computations) |
![]() | FLOW LINES | Defines logical sequence of the program. It points to the next symbol to be performed. |
![]() | ON-PAGE CONNECTOR | Connects to the flowchart to avoid spaghetti connection on the same page. |
![]() | OFF-PAGE CONNECTOR | Connects the flowchart on different page to avoid spaghetti connection. |
![]() | DECISION | Process conditions using relational operators. Used for trapping and filtering data. |
Symbols used in flowchart representation.
7. Guidelines to follow
Following guidelines should be applied while designing a flowchart.
- The flow of the Flowchart should be from top to bottom.
- If the problem is complex one, make use of connecting blocks to simplify the problem.
- Intersecting flow lines should be avoided.
- Meaningful descriptions should be used in the symbols.
Algorithm Assignment Question