Creating a program that will read from a file
Use Eclipse Java program!!!
Concepts tested by this program:
- Create a Comparator
- Create a TreeSet using a comparator
- Collections.sort using a comparator
- Use a generic class
- JTable – select a row
You will be creating a program that will read from a file. For each sentence, the word with the largest Scrabble value and the Scrabble value for that word will be displayed.
The Scrabble values are as follows:
- 1 point: E, A, I, O, N, R, T, L, S, U
- 2 points: D, G
- 3 points: B, C, M, P
- 4 points: F, H, V, W, Y
- 5 points: K
- 8 points: J, X
- 10 points: Q, Z
Comparataor - ScrabbleComparator
Create a ScrabbleComparator that implements Comparator<String>. The compare will sort the strings in reverse order by Scrabble Value. The largest value will be first.
Data Structure - TreeSet. Construct the TreeSet with the ScrabbleComparator. Use for getLargest. All words are added to the TreeSet. The largest is retrieved with the first method.
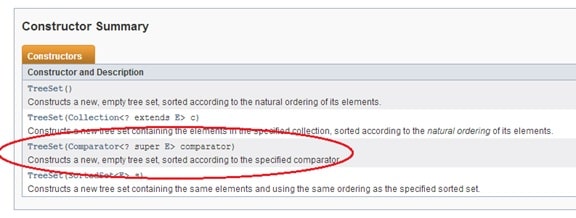
Data Manager/Utility classes
- Create a ScrabbleUtility class with the following static methods:
- String getLargest(String). Determines the largest scrabble value of words in a Sentence and returns the word (String) with the largest scrabble This method will store the words of the string into a TreeSet constructed with the ScrabbleComparator.
- TreeSet getLargest(String, int). This method will store the words of the string into a TreeSet constructed with the ScrabbleComparator and return the TreeSet This method is for testing purposes only.
- int getScrabbleValue(String). Determines the scrabble value of the string and returns the value.
- ArrayList<Pair<String, Integer>> getScrabbleValues(String). Determines the scrabble value of each word in the String and returns an ArrayList of Pairs. Each Pair contains the word(a String) and the Scrabble value (an Integer). Use the Pair<T,S> class provided. The first word in the string will be represented by the first Pair in the ArrayList. The fifth word in the string will be represented by the fifth Pair in the ArrayList.
- ArrayList<Pair<String, Integer>> sortScrabbleValues(String). Determines the scrabble value of each word in the String, sorts them in Scrabble Order and returns an ArrayList of Pairs. Each Pair contains the word(String) and the Scrabble value (Integer). Use the Pair<T,S> class provided. Use the Collections.sort with the comparator that sorts in Scrabble Order.
The GUI
If the user selects the Read Text File button, use the JFileChooser to ask the user for the location of the text file to be read and then display the contents of the file in a table. Use a table so that a row of the table can be selected.
If the user selects the Largest Scrabble Value button, display the largest word and the scrabble value for each sentence.
If the user selects a row in the table and the All Scrabble Values in Sentence button, display all the words in that sentence with their scrabble values. If the user selects the Sort in Scrabble Order button, the words of the sentence and their scrabble values are displayed with the largest scrabble value word first.
You can assume that the files are correct and there is one sentence per line.
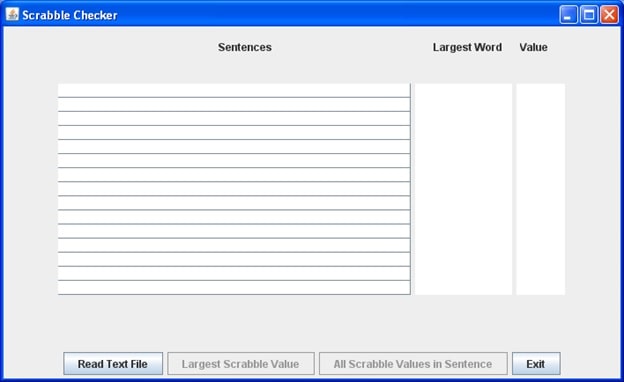
Disable Largest Scrabble Value button until user Selects Read Text File Button.
Read Text File
Use JFileChooser to allow user to select text file. Read from text file and place into Table.
Enable the Largest Scrabble Value Button
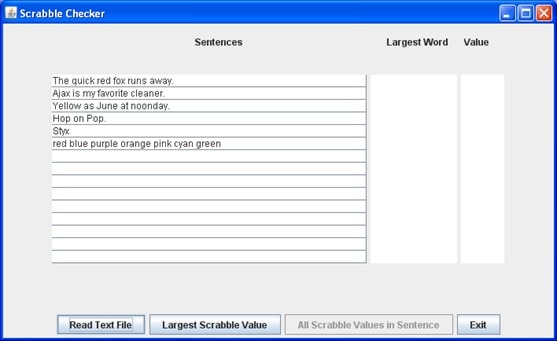
Disable All Scrabble Values in Sentence button until user selects a row in the table.
Results of Largest Scrabble Value:
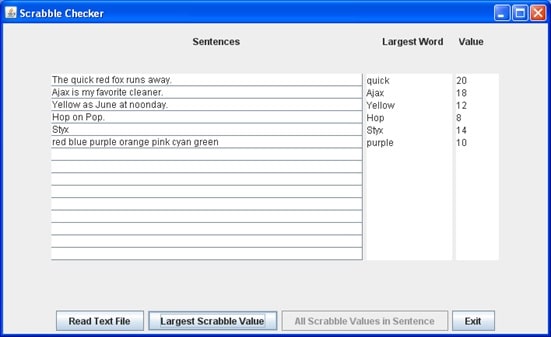
When user selects a row in the table, the All Scrabble Values in Sentence button becomes enabled
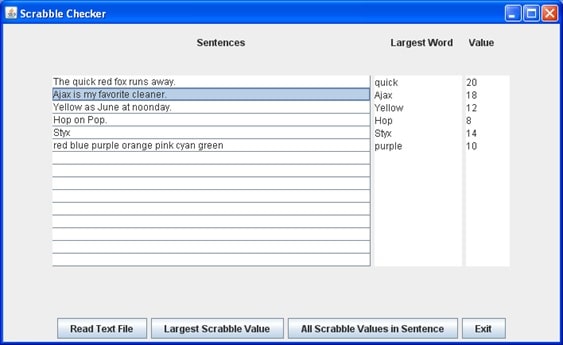
Results of All Scrabble Values in Sentence:
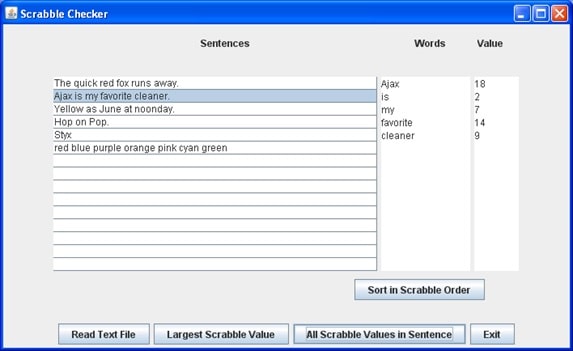
Sort in Scrabble Order button becomes visible.
Results of Sort in Scrabble Order:
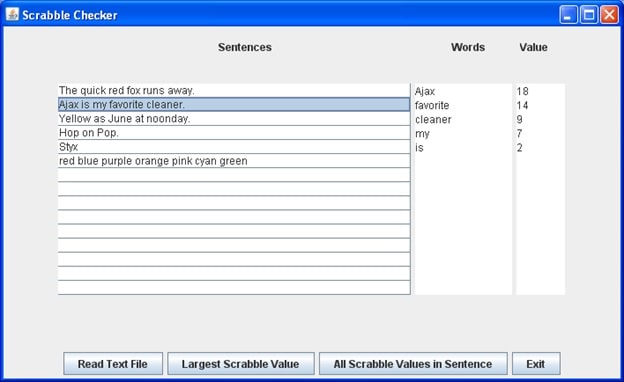
DOCUMENTATION
Javadoc for user created classes: 4 pt____
Test Cases 4 pt____
JUnit Test Class
Implement the student test methods of the ScrabbleUtility
UML Diagram 2 pt ____
PROGRAMMING
Programming Style
Internal class documentation (within source code) 5 pt ___
Class description using Javadoc
Author’s Name, Class, Class Time
Methods commented using Javadoc
Program user interface
Clear to user how data is to be entered 1 pt____
Output is easy to understand 1 pt____
Accuracy
Public tests (those I gave you and your additions) 5 pt ____
Private tests 5 pt ____
Program Details
- ScrabbleComparator class 6 pt _____
- Implements Comparator<String>
- Sorts in reverse order on Scrabble Value
- ScrabbleUtility class 10 pt _____
- getLargest – uses TreeSet and Comparator
returns the largest String in the TreeSet
- getScrabbleValue – returns the scrabble value for a string
- getScrabbleValues – returns pairs of words and scrabble values
for each of the words in the string
- sortScrabbleValues - uses Collections.sort and Comparator to sort
by ScrabbleValue, returns pairs of words and scrabble values for
each of the words in the string
- GUI classes 7 pt _____
- Uses FileChooser to select text file
- Reads from a file and puts contents in a table
- Uses the methods of the ScrabbleUtility
- Buttons are enabled when appropriate
- User can select one row of the table
Total 50 pt _____
Comments: