CMIS 102 Hands-On Lab Week 8
Week 8 - Concerts Overview
This hands-on lab allows you to follow and experiment with the critical steps of developing a program including the program description, analysis, test plan, and implementation with C code. The example provided uses sequential, repetition, selection statements, functions, strings and arrays.
Program Description
Compute the value of the ticket sales for concerts and the total sales for a series of concerts. Then display the concert information in a sorted list.
Technologies used in this project:
- strings stored as char arrays
- 2-dimensional char and int arrays
- getting data from the user into the various arrays
- using only part of an array for valid data
- computing array arithmetic – vector inner products to compute a value
- sorting using selection sort
- displaying data in arrays Interaction
Outline:
- Get the ticket price for each 3 categories.
- float
- Get the band name and numbers of fans for each category of each concert. a. String int int int
- NOTE: spaces are not allowed in the band names, so use underscores instead of spaces. c. Period will end the input.
- Internal computations:
- Compute the value of the sales for each concert.
- Compute the total value of the ticket sales.
- Sort the concerts by value of the ticket sales.
- Display the resulting data in a nice format.
Notes:
- This program is not expected to behave nicely if the input does not follow the specifications above.
- All numbers this program will handle should be of moderate size. The exact meaning of moderate is made precise by the data types, print statements, and array sizes.
Analysis
- Since we are going to do sorting, we will need to hold the data in arrays.
- We will use functions to simplify the code and reflect a modular approach to the design of the project.
- We will use global variables to reduce the amount information transferred in parameter lists.
- We will use a fixed number of categories but let the number of concerts by determined by the user at run time, up to a maximum value.
- The user will end inputting the group information with a flag value, a period.
- Basic data types
- ticket prices – float
- name of concert/band – char array
- number of concerts and number of fans in each category at each concert – int
- number of concerts – int.
- value of tickets – float
- We will use nested loops to handle most operations:
- reading the ticket prices
- reading the group name and attendance
- computing the value of the ticket sales
- printing the array of data
- sorting the arrays by the value of the ticket sales
Pseudo-code:
Global variables:
- Constants using define declarations o MAXN – the maximum number of characters in a band name o MAXG – the maximum number of concerts/bands/groups o MAXC – the maximum number of categories Arrays:
- char group [MAXG][MAXN] – the name of each group
- int fans [MAXG][MAXC] – the number of fans at a concert by category o float prices [MAXC] – the ticket price of each category
- float sales [MAXG] – the total value of the tickets for each concert
- int count o the actual number of active concerts/bands/groups o this number is determined at run time in the getData function
- used throughout the rest of the program to only look at data in the group, fans and sales arrays that is actually active.
Pseudo-Code:
- main
- getData – get ticket prices by category & get data for the concerts
- computeSales – compute sales for each concert
- printArray – print raw concert data
- sortBySales – sort the concerts by value of sales, use selection sort
- findMinSales – find min sales in unsorted rest of array
- switchRows – as needed
- printArray – print sorted concert data
- getData
- prompt for ticket prices
- get ticket prices into prices array
- prompt for band name and fans in each category at each concert
- insert data into appropriate arrays, ending with a period
- loop on concert
- get group name
- loop inside on number of categories
- computeSales
- for each group
- sales for that group = 0
- for each category
- sales = sales + price of category * number of fans in that category
- for each group
- loop on concert
- printArray
- print column headers
- for each group
- print the group name
- for each category
- print the number of fans in that category iii. print the sales for that concert/group
- sortBySales
- COMMENTS: for each row (group + fans + sales)
- for each row index, starting at index 0
- the rows that have indices less than the current index are sorted, and are already the smallest sales elements in the entire data set.
- COMMENTS: for each row (group + fans + sales)
- thus, the next step is to find the smallest sales row in the remaining elements and switch that row with the current index row
- for index = 0 to number of active rows, step index by 1
- target = findMinSales (index) ii. if target not equal to index, switchRows (target, index)
- findMinSales (index)
- set target as index
- min
- for the rest of the array, starting at index+1, indexing by i
- if sales [i] < min
- target = i
- min = sales [target]
- return target
- if sales [i] < min
- switchRows (m, n)
- Temporary locations
- char tc
- int ti
- Temporary locations
- float v
- switch group names by stepping through all the letters (i) in each char array:
- tc = group [m][i]
- group [m][i] = group [n][i]
- group [n][i] = tc
- switch fan entries, stepping by (i) over the fans array:
- ti = fans [m][i]
- fans [m][i] = fans [n][i]
- fans [n][i] = ti
- switch sales:
- v = sales [m]
- sales [m] = sales [n] iii. sales [n] = v
Test Plan
To verify this program is working properly a variety of test cases should be used.
The following is just one test case, which only tests a very few aspects of the program.
You should be thinking about other data sets to test other aspects of the program, and also create more realistic test sets.
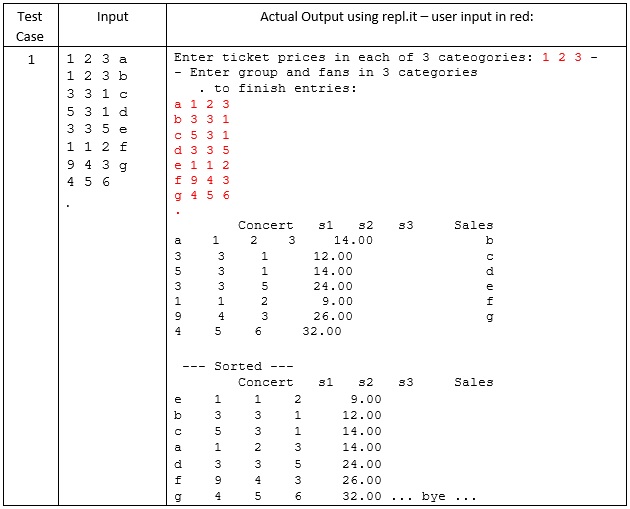
Coding Plan
These are the steps taken to develop the base code of this project.
You should start with this code to address the Learning Exercises below.
This is a rather complex project, and it should be developed in SMALL steps, making SMALL changes to the code and testing each change carefully before moving on to the next step. Since we have a pretty good pseudo-code representation of this project, and a good test plan, we should be able to develop the steps we think this project will require.
We are guided here by the requirements of our environment, particularly getting the C compiler to accept our code at each step without error messages.
It is also helpful to have some idea of how long each step should take, so that if our actual time trying to complete a step is getting out of hand, we know it is time to change our strategy for that step by, for example:
- taking a break,
- looking for more specific information (don’t take too long here either!),
- asking someone (your instructor is the best choice) for help!
Task |
Description |
Notes |
1 |
Hello world |
Get the basic environment working Get main method started Even change the message to Bye |
2 |
#define stuff |
These tokens will be used in the array declarations, and throughout the code Getting the syntax of these declarations correct can be a challenge |
3 |
Declare the arrays And count |
The global variables this program will use. Get this syntax to work, and work with the define declarations |
4 |
getData function |
Just get the declaration to compile correctly |
5 |
Call getData in main |
Making sure we have the call syntax correct |
6 |
Other function declarations |
As specified in the pseudo-code. Again, we want to check with the compiler that we are using the correct syntax |
7 |
TEST CODE: count = 1 values to one group |
Give values for one concert in main: count = 1; following requires #include <string.h> strcpy (group [0], "one"); fans [0][0] = 3; fans [0][1] = 12; fans [0][2] = 33; sales [0] = 23.23; |
8 |
printArray |
Call this function from main. Focus on getting this function working Check the output for each step. Lots of steps:
|
9 |
More test code |
Create another set of code like step 7 to test printArray more carefully |
10 |
Call getData in main |
Start with a printf statement saying “got here” in getData |
11 |
Print prompt |
For ticket prices of 3 categories, remove “got here” message |
12 |
Get ticket prices |
Use for loop to get prices into sales array Add for loop to print the values in that array – temporary code |
13 |
Get group loop |
For loop to get group name (index is i) Read group [i] Add test for name is period and break input loop if so TEST the period |
14 |
Get fan numbers |
Nested for loop over the number of categories (index is j) Read fans [i][j] Increment count since this should be a valid group Temporary: set sales [i] to 44.56 or some other random number |
15 |
Fix main |
Comment out the test code from steps 7 and 9 Insert the appropriate calls in main: getData (); printArray (); Test the code with real data! |
16 |
computeSales |
Get this code working, using a loop Add the call to this function to main |
17 |
Sorting |
Get the call to sorting active Add the print statement to say the following is sorted Print the “sorted” array (not sorted yet) |
18 |
findMinSales |
Get this code working – starting at an index, find the index of the smallest sales in the rest of the list Use print statements to make sure this is working |
19 |
switchRows |
Get this working – switch group name, the fans and the sales values |
20 |
Final check |
Try a number of different data sets here. |
C Code
The following is the C Code that will compile in execute in the online compilers.
{` // C code // This code will compute the values of the sales ticket sales for concerts // and sort the entries by those values // Developer: Faculty CMIS102 // Date: Jan 31, XXXX #include < stdio.h> #define MAXN 100 // max characters in a group/concert name #define MAXG 50 // max concerts/groups #define MAXC 3 // max categories char group [MAXG][MAXN]; int fans [MAXG][MAXC]; float prices [MAXC]; float sales [MAXG]; int count = 0; void printArray () { printf ("%15s%5s%5s%5s%10s\n", "Concert", "s1", "s2", "s3", "Sales"); for (int i = 0; i < count; i++) { printf ("%15s", group [i]); for (int j = 0; j < MAXC; j++) { printf ("%5d", fans[i][j]); } // end for each category printf ("%10.2f\n", sales [i]); } // end for each group } // end function printArray void computeSales () { for (int i = 0; i < count; i++) { sales [i] = 0; for (int j = 0; j < MAXC; j++) { sales [i] += prices [j] * fans [i][j]; } // end for each category } // end for each group } // end function computeSales void switchRows (int m, int n) { char tc; int ti; float v; // printf ("Switching %d with %d\n", m, n); for (int i = 0; i < MAXN; i++) { tc = group [m][i]; group [m][i] = group [n][i]; group [n][i] = tc; } // end for each character in a group name for (int i = 0; i < MAXC; i++) { ti = fans [m][i]; fans [m][i] = fans [n][i]; fans [n][i] = ti; } // end for each fan category v = sales [m]; sales [m] = sales [n]; sales [n] = v; } // end switch int findMinSales (int m) { float min = sales [m]; int target = m; for (int i = m+1; i < count; i++) if (sales [i] < min) { min = sales [i]; target = i; } // end new max found return target; } // end function findMinSales void sortBySales () { int target; for (int i = 0; i < count; i++) { target = findMinSales (i); if (target > i) switchRows (i, target); } // for each concert } // end function sortBySales void getData () { // for (int i = 0; i < MAXG; i++) sales [i] = 0; printf ("Enter ticket prices in each of %d cateogories: ", MAXC); for (int i = 0; i < MAXC; i++) scanf ("%f", &prices [i]); printf ("-- Enter group and fans in %d categories\n", MAXC); printf (" . to finish entries:\n"); for (int i = 0; i < MAXG; i++) { scanf ("%s", &group[i]); if (group [i][0] == '.') break; count++; for (int j = 0; j < MAXC; j++) scanf ("%d", &fans[i][j]); } // end for each group } // end function getData int main(void) { getData (); computeSales (); printArray (); printf ("\n --- Sorted ---\n"); sortBySales (); printArray (); printf("... bye ...\n"); return 0; }`}
Learning Exercises for you to complete
- Demonstrate you successfully followed the steps in this lab by preparing screen captures of you running the lab as specified in the Instructions above.
- Add a function to print welcome information, including your name and an introduction to the project, and, of Assignment, call this function as the first instruction in main.
NOTE: It is convenient to put each test case into its own data file so you don’t have to type a rather large set of input over and over again. Then you can just copy/paste the data into the interaction panel of the IDE you are using.
- Modify the program to add a function to compute and display the total sales for all the concerts. Support your experimentation with screen captures of executing the new code.
- Enhance the program to allow the user to enter 4 categories.
Explain the changes you made to the code, and create appropriate test data files. Support your experimentation with screen captures of executing the new code.
- Prepare a new test table with at least 2 more test cases listing input and expected output for the new code you created, supporting 4 categories.
- Create a variety of test cases focusing on the sorting algorithm, such as the final element is the smallest, the entire set is already sorted, etc.
Explain the purpose of each test case, and check your code against each of those cases.
- Try using different inputs:
- What changes would you suggest to handle larger, more realistic numbers?
- What happens if any of the numbers, such as the ticket prices, are negative?
- What are your recommendations concerning negative input values?
- What changes should be made to the code if the customer wished to sort on the number of fans in category 1, the first of the three (or four) categories?
Make those changes, test your code and confirm that it is working correctly.
Grading guidelines
Exercise |
Submission |
Points |
1 |
Demonstrates the successful execution of this Lab within an online compiler. Adds and calls a function to display introductory information. Provides supporting screen captures. |
10 |
2 |
Modifies the code to add a function to sum each concert. Supports your experimentation with screen captures of executing the new code. |
10 |
3 |
Modifies the code to handle 4 categories, with explanation. |
10 |
4 |
Prepares at least 2 additional test cases for 4 categories. |
10 |
5 |
Prepares test cases focusing on sorting algorithm. Supports your experimentation with screen captures of executing the new code. |
15 |
6 |
Documents experiments with unusual but valid inputs. |
10 |
7 |
Modifies the code to sort on category 1. Creates appropriate test cases. Documents results showing correctness of modifications. |
15 |
8 |
Document is well-organized, and contains minimal spelling and grammatical errors. |
20 |
Total |
100 |